題目:
Given a binary tree, each node has value 0
or 1
. Each root-to-leaf path represents a binary number starting with the most significant bit. For example, if the path is 0 -> 1 -> 1 -> 0 -> 1
, then this could represent 01101
in binary, which is 13
.
For all leaves in the tree, consider the numbers represented by the path from the root to that leaf.
Return the sum of these numbers.
範例:
Example 1:
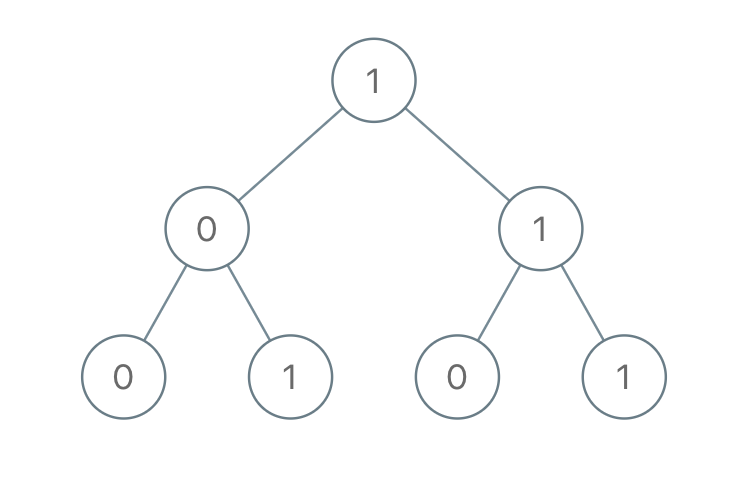
Input: [1,0,1,0,1,0,1]Output: 22Explanation: (100) + (101) + (110) + (111) = 4 + 5 + 6 + 7 = 22
解法:
這題一開始知道要用遞迴寫
因為要有辦法計算父節點的值
但題目給的func不能符合需要
所以要再自己寫一個func
直到最後一個節點才需要計算值
所以func的計算會有3種
1. 當前節點等於null
2. 當前節點是終端節點
3. 要遞迴子節點計算
程式碼:
/**
* Definition for a binary tree node.
* type TreeNode struct {
* Val int
* Left *TreeNode
* Right *TreeNode
* }
*/
func sumRootToLeaf(root *TreeNode) int {
if root == nil {
return 0
}
sum := iter(root, 0)
return sum
}
func iter(root *TreeNode, val int) int {
if root == nil {
return 0
}
val = val << 1 + root.Val
if root.Left == nil && root.Right == nil{
return val
}
return iter(root.Left, val) + iter(root.Right, val)
}
沒有留言:
張貼留言